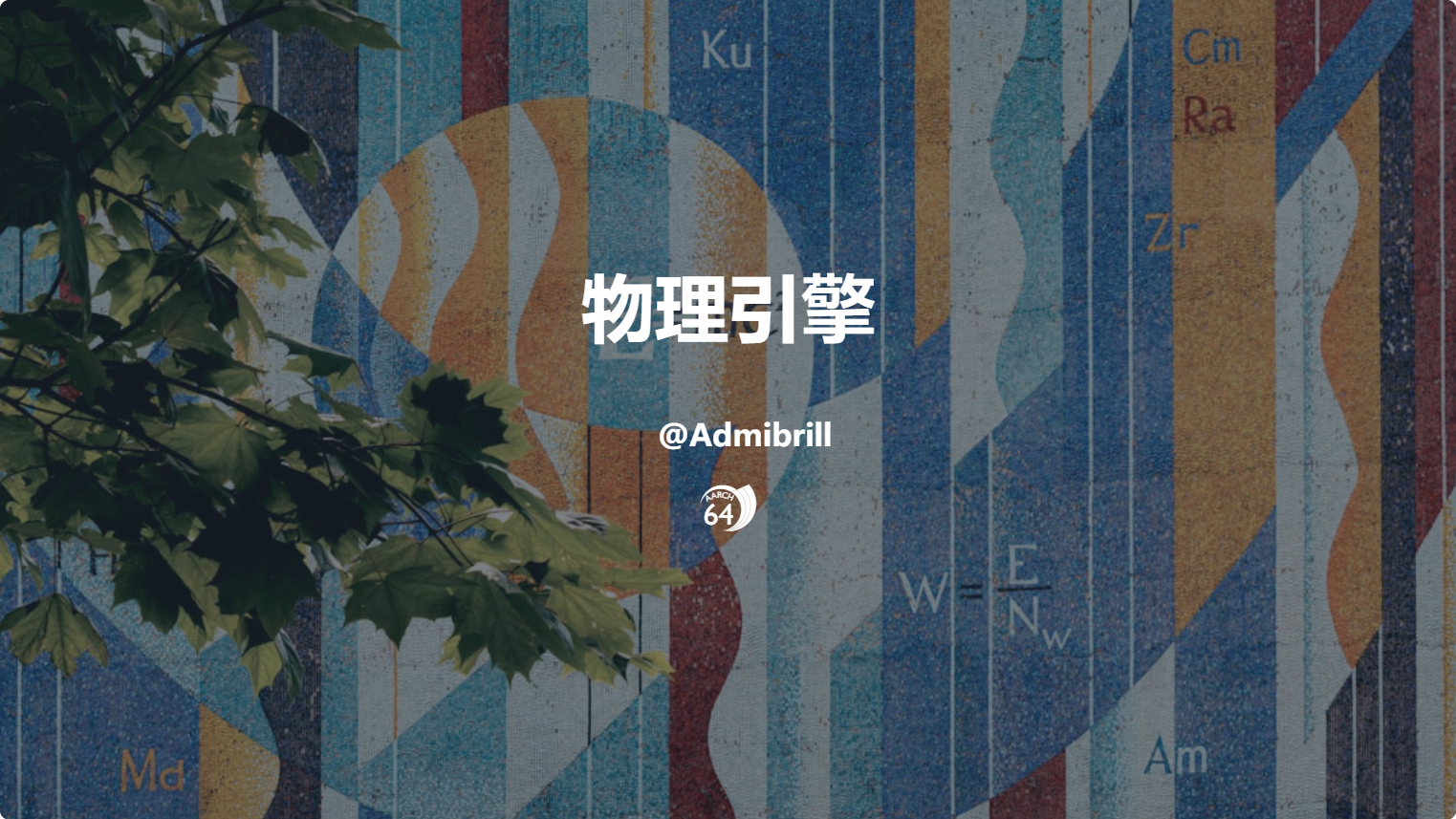
自己动手写二维物理引擎(一)
前言
这一篇,继续之前的想法,开始写物理引擎的代码。
由于pygame不能同时打开多个窗口,我决定换Qt来显示窗口。
开始
导入库
打开physics.py
1 | from PySide6 import * |
PySide6
:这是Qt官方的PyQt6的库。这里我们用它来实现窗口以及内容的显示。math
:Python数学库,用来处理一些高级的物理计算。
全局常量
1 | DYMANIC=0 |
这些常量定义了物体的状态等信息,后学会有更多常量写在这里。
Qt应用程序
1 | qapp=QtWidgets.QApplication([]) |
之后在demo.py
中运行physics.run()
即可运行应用程序。
物理空间
编写一个Space(QtWidegets.QWidget)
类来模拟一个物理空间,这将被显示为一个窗口。
实例化
1 | class Space(QtWidgets.QWidget): |
width
:窗口的宽度height
:窗口的高度caption
:窗口的标题objects
:物理空间中所有物体列表g
:重力加速度(常量)timer
:使用qt计时器,用来定时运行一些函数
启动窗口
1 | # class Space(QtWidgets.QWidget): |
该函数可以使物理空间窗口弹出并初始化。
移动与绘制
一个使窗口移动,并制造重力,另一个绘制。
1 | # class Space(QtWidgets.QWidget): |
力与物体对象
力
力是最简单的一个类,它是一个向量,只有两个成员:方向和大小。
特别注意,所有的角单位都用弧度($\pi\space \text{rad}=180^{\circ}$)
1 | class Force(): |
物体对象
物体对象有很多种,如刚体、流体、关节
实例化
1 | class Object(): |
z
:层坐标,z
不同的两个元素不会发生碰撞。window
:所在的窗口color
:对象显示的颜色mass
:对象的质量velocity
:运动速度angle
:运动方向
人为施力
可以看出,我们可以利用三角函数把物体当前的方向和速度分解为水平方向上的速度和垂直方向上的速度,对施加的力作同样的处理,将水平和垂直的分别相加再转换为新的速度和角度就可以实现人为施力。
于是又了下面的代码:
1 | # class Object(): |
删除对象
把对象移除,不多赘述
1 | # class Object(): |
刚体
目前先实现圆、线段。之后会实现多边形。
1 | class Solid(Object): |
angle
与direction
的区别
angle
是指运动方向。而direction
是只物体本身的朝向。
效果测试
demo.py
1 | import physics |
下期预告
下一期会实现多边形以及碰撞检测。886!
本文是原创文章,采用CC BY-NC-SA 4.0协议,完整转载请注明来自Admibrill
评论 ()